Setting up Python on Windows with Anaconda
05 Mar 2019
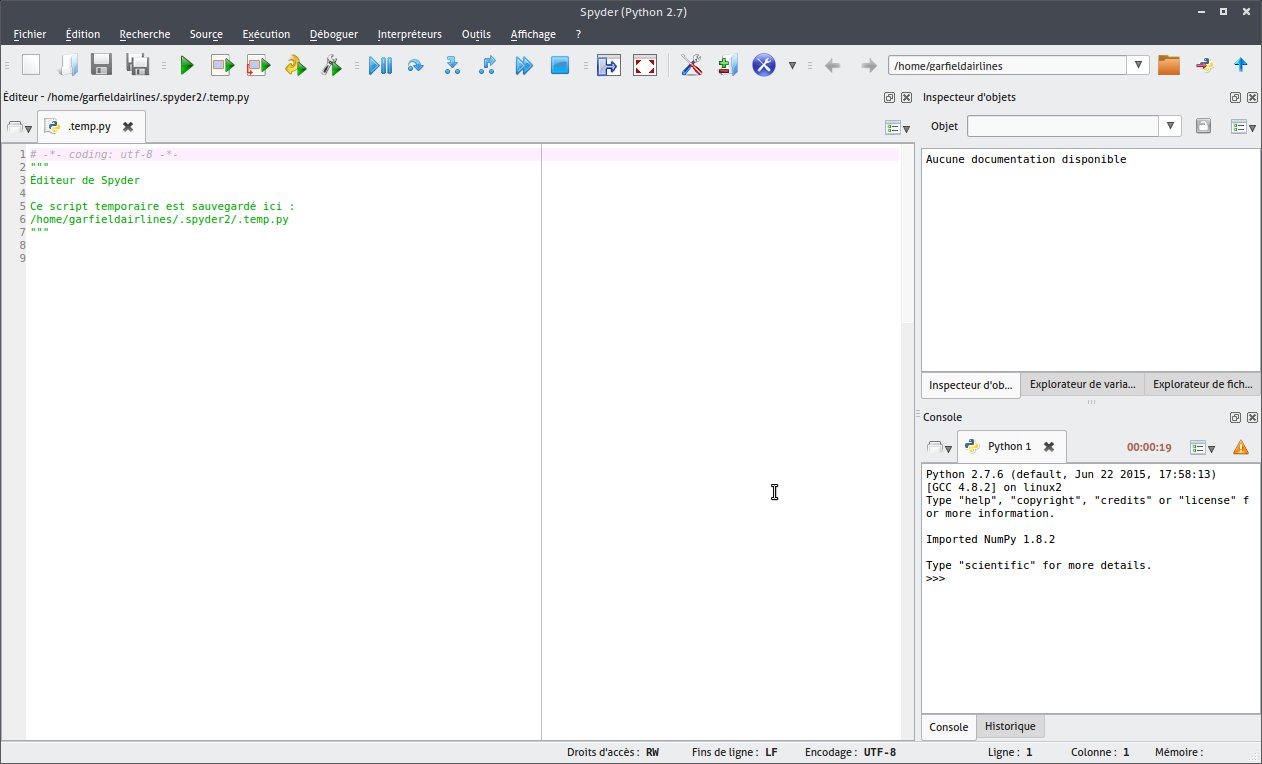
I keep warning students not to use real, corporate CSV files in Python with online “IDEs” like Repl.it or CodeBunk, and to install Python on your local machine instead.
Finally, I’ve written a tutorial to walk you through it!
These screenshots document the simplest approach I know of for installing Python on a Windows machine.
Seriously – THIS IS SO SIMPLE. You just download software, double-click the installer, let it run, open up the code editor, and execute your first print('Hello World')
like magic.
But I’m here to walk you through every step and explain what’s going on in the background.
If things don’t work and you can handle something a bit more complicated, see my instructions on setting up “miniconda” on Windows.
(Alternatively, I wrote a tutorial on installing the Windows Store version of Python.)
Did you get Anaconda installed?
Yay! I’m thrilled I could help.
If you’d like, I’d love a Ko-Fi. (Chai for me!) 🥰
Installing Anaconda & running a Python program
Download
First, download an appropriate installer file for “Anaconda”.
It’s not very smart about detecting that you’re on Windows, so explicitly click the “Windows” option just above the downloads.
I recommend getting a 64-bit version, as long as your computer is 64-bit.
The only reason I can think of to have a 32-bit version on your computer is if, say, you’re going to be connecting to an Oracle database using “Oracle Client” software already installed on your computer, with that software being 32-bit (e.g. because the database itself requires 32-bit clients).
Or, I suppose, if for some weird reason you have a 32-bit computer. But then you’re probably worried about “bloat” in things you install and would want to follow the more difficult miniconda instructions anyway.
If what I just said didn’t make any sense, do 64-bit.
You want one for “Python 3.something,” not “Python 2.something.”
Run the Anaconda installer
Double-click the “.exe” file to run setup. Click “Next,” then click “I Agree” on the following screen.
Choose “just me” or “all users”
I’m going to install this for “just me,” because I don’t have admin rights to install software on my corporate PC.
My choice here influences whether the installer suggests installing the program under:
C:\Users\MY_USERNAME\AppData\Local\Continuum\anaconda3
(just me), orC:\Program Files\Anaconda3
(all users)
Installation folder
It bothers me to forget when software is installed in my \AppData\Local\
hidden folders, so I am going to manually change the installation path on the next screen to C:\Users\MY_USERNAME\Documents\ProgFiles\Continuum\anaconda3
.
(Yes, in the screenshot, which I reused from an earlier tutorial, I called that folder “ProgramFilesForSoftwareHatingSpaces
” instead of “ProgFiles
” – I was feeling a bit pouty when Anaconda complained about my “Program Files” folder I’d set up under “My Documents.”)
Options
Next, the installer is going to ask me whether I want to do either of two things.
- Add “Anaconda” to my “PATH” environment variable. It says not to do it right in the installer text, so I’m going to leave it un-checked.
- Register “Anaconda” as my “default Python 3.7” environment. This comes checked, and they recommend it, and hey … let’s do it. I did.
Now I’ll click “Install.”
While it runs
The installer takes a few minutes while it dumps thousands of small files onto your hard drive.
Q: Why so many files?
A: Your computer doesn’t naturally understand Python commands.
At a really low level, it understand commands in its “assembly language” (which you wouldn’t want to have to write by hand).
All Windows computers are shipped understanding a language called “C” (which, if you don’t want to become a professional programmer, you probably still wouldn’t want to have to write by hand).
Lots of support programs written in “C” teach your Windows computer how to understand commands written in “Python” (presuming you, or a program like an “IDE” acting on your behalf, tell Windows where exactly you installed your software that can help parse these commands – for me, that would be C:\Users\MY_USERNAME\Documents\ProgFiles\Continuum\anaconda3\python.exe
).
Furthermore, the full version of Anaconda comes with a lot of Python “libraries” / “packages” / “modules” (extensions to the base set of Python commands you can program with), all of which also have to have files on your computer so that your computer can understand what they mean.
If you don’t want so many of these, you could always follow the more difficult instructions on setting up “miniconda” on Windows instead.
Finishing
When it says “Completed”, click “Next.”
Don’t bother to install VSCode – it’s an “IDE” (code editor), but you’re already getting another one called “Spyder” with this installation, and that will do you just fine. Click “Skip.”
Leave the “learn more” boxes checked, or un-check them, as you prefer, and click “Finish.”
(The second checkbox brings you here; the first just brings you to Anaconda’s website.)
Write your first Python program
If you’ve practiced coding in an online “IDE” like Repl.it or CodeBunk, you know that coding can be as easy as clicking a big “run” button every time you type enough text that you wonder what it does.
You have the same thing on your hard drive now! It’s called Spyder.
Anaconda’s installer installed it for you.
If you look at your “Start” menu (the thing that pops up when you click the Windows icon in the bottom left corner of your screen), you may also notice a new folder called “Anaconda3 (64-bit)” with several icons beneath it.
Click the one that says “Spyder.”
The splash screen while it starts up looks like this:
And in your taskbar, once it’s up and running, its icon looks like this:
When it’s fully open, it’ll typically look something like this:
- On the left will be a place for you to edit code, with a new file called “untitled.py” (tentatively proposing you save it in
C:\users\YOUR_USERNAME\untitled0.py
) whose contents are:
# -*- coding: utf-8 -*-
"""
Created on SOME_TIMESTAMP
@author: YOUR_USERNAME
"""
On the right, or at the bottom right, will be some sort of “console” where you can watch code execute every time you hit the big green “run” button near the top of the screen (it’s a right-facing green triangle).
In the top menu, click “File” -> “Save As.”
Pick a nice folder for your program (personally, I’m going to save mine under C:\example\
, but you might want to put it on your desktop) and save it there with a filename of “hello.py
”.
(Spyder’s “run” button complains if you try to run code before giving it a proper spot on your hard drive. You could just do file->save, but I personally can’t stand cluttering my c:\users\MY_USERNAME\
with files like “untitled0.”)
(Note that in the photo, mine is called “hello2.py
” because I reused a screenshot from a different tutorial with 2 steps!)
Now erase the entire contents of the file, and on line 1, type:
print('Hello world')
Then click the big green “run” button.
You may see a pop-up dialog with all sorts of options about executing code consoles or interpreters and such.
Just click “cancel” on the pop-up dialog box and then click the play button again.
The pop-up box was giving you a chance to highly customize where you want to see any output from running your code.
Clicking “cancel” and trying again seems to tell Spyder, “you figure out where to display the output of this code, don’t bother me with all these options.”
(Unfortunately, I didn’t catch this dialog box with a screenshot – Spyder seemed to remember my settings from my previous usage of it with “big Anaconda.”)
Your output should look something like this – somewhere at the right-hand side of the screen, probably in a console called “Console 1/A,” you’ll see confirmation of the filepath of “hello.py
,” followed by a new line with the text “Hello World
”.
(Note that in the photo, mine says “Hello again
” because I reused a screenshot from a different tutorial with 2 steps!)
🎉🎊🙌
Congratulations – you’ve written and run a Python program!
🎈🎆👍🏾
In the top menu, click “File” -> “Save” because why not be proud of this working code? It’s good to get used to saving your work as soon as you like it.
But before we write a bigger program, let’s take a little diversion to learn about “modules.” They’re pretty important in Python.
Modules
Checking whether “modules” are installed
Now you need to learn what it looks like when a given extension to the Python language, also known as a “library” or “package” or, particularly in Python, a “module,” is installed.
Backspace out the entirety of your code and on line 1, type:
import pandas
- Trivia: the orange warning triangle to the left of the line numbers in your code is telling you that you “imported” a package that your program doesn’t actually use anywhere else in the program. That’s Spyder trying to help you write better, more efficient code.
- Red circles with “x” in them to the left of the line numbers means a line doesn’t even contain legitimate Python to begin with. Those, you’ll want to care about, or your program won’t run.
Yay, it exists!
Click the “run” button.
If you do have the “pandas” module installed, nothing special happens.
You should have it installed – it comes with the “big”/mainstream version of Anaconda. Modules like Pandas is why Anaconda took so long to install!
If you have “pandas” installed, when you run this code, you just get another command prompt (“In[SomeNumber]
”) in the box at the right where the number in square brackets is 1 bigger than it was before.
(Note: in the screenshot above, things look a slightly different because I’m still sooooo lazy and reusing screenshots from a different tutorial – sorry!)
Boo, it doesn’t exist!
Let’s try “importing” a module you probably don’t have, because it doesn’t come with Anaconda.
Backspace out the entirety of your code and on line 1, type:
import simple_salesforce
Click the “run” button.
If you get a huge dump of colorful text in your console, with the last few lines of the big error message saying the following, you now know that you don’t have the module called “simple_salesforce
” installed:
exec(compile(f.read(), filename, 'exec'), namespace)
File "C:/example/hello.py", line 1, in <module>
import simple_salesforce
ModuleNotFoundError: No module named 'simple_salesforce'
Installing a “module”
Anaconda comes with just about everything you need as a total beginner, so don’t bother installing extra “modules” on your computer just yet.
However, if you ever need any, see the “modules” section of my instructions on setting up “miniconda” on Windows – people who install “miniconda” have to install all their own “modules” by hand, so I went over it step-by-step in those instructions!
Write your second Python program
Backspace out the entirety of your code.
Copy and paste the following code into your code editor and run it:
import pandas
pandas.set_option('expand_frame_repr', False)
filepath = 'https://raw.githubusercontent.com/pypancsv/pypancsv/master/docs/_data/sample1.csv'
df = pandas.read_csv(filepath)
print(df)
You should see the following output in your console at right:
Id First Last Email Company
0 5829 Jimmy Buffet [email protected] RCA
1 2894 Shirley Chisholm [email protected] United States Congress
2 294 Marilyn Monroe [email protected] Fox
3 30829 Cesar Chavez [email protected] United Farm Workers
4 827 Vandana Shiva [email protected] Navdanya
5 9284 Andrea Smith [email protected] University of California
6 724 Albert Howard [email protected] Imperial College of Science
Now you’ve opened a CSV spreadsheet file with Python – you’re off to the races!
Head here to learn new skills and put them in action on your computer.
Happy Programming
You now have a working environment for editing CSV files in Python!
- You have an “IDE,” or as I like to think of it, a “text editor with a run button,” called Spyder, that runs code you type into it as you see fit.
- You know where to look to learn to install & update “modules” that you hear might be useful.
(Be careful and don’t trust any old module you find on the internet. It’s nothing more than “someone else’s code that you’re choosing to run on your computer.” Make sure it’s not from someone sketchy.)