Logging into Salesforce's Pardot API (w/ Python)
04 Apr 2019
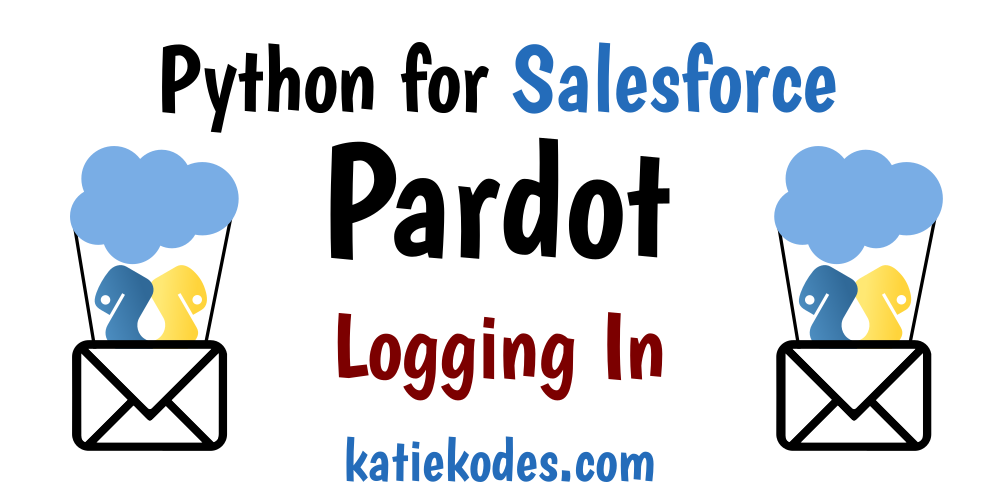
Logging into Pardot’s API is a lot easier than logging into MarketingCloud’s API was.
I used Python, but most of the steps have nothing to do with code.
Any language that can do HTTPS POST requests will do.
Background
I love “automating the boring stuff” by programming against Pardot’s API.
Two common things I do are:
- Add a lot of custom fields to the Prospect table at once
- Clean up a lot of bad data at once
Password Precautions
To get this code to work, you have to type your Pardot password and “user key” into your code. That’s not a good thing. Anyone who intercepts this information could imitate you in Pardot.
- Only run this code on a computer you trust.
- Only run this code over a network connection you trust.
- If you save this Python script in a file, type “CONTAINS PASSWORD” at the beginning of the filename so you’ll remember it’s a file in which you have to clear out passwords before you close it.
- If you’re working in an IDE that treats subsequent “run” button clicks like one big session (and caches the values of variables from “run” to “run”), leverage that to help you remember to clear out your password from your file. Run a few lines of code, with the rest commented out, to fetch an “API key” and save it into a variable called
api_key
.
Then comment out the “fetching” code, backspace over your password, type the word “PASSWORDGOESHERE
,” and save your script.
Do the same with your e-mail address and user key.
Ta da! Now you can stop worrying about whether you left a password in plain text in the contents of your script. - You’re going to have to get expert advice if you need to store this script and run it in any sort of automated fashion. Typing your password into the body of the script itself and leaving it there is simply not an option.
1: Admin -> User Management -> Users
Log into Pardot’s web console as a system administrator.
If the left navigation menu isn’t already expanded, click the navigation “hamburger” (icon with three horizontal lines) at the top of it.
Hover over “Admin,” then hover over “User Management,” then click “Users.”
You can also get directly to this screen by simply visiting https://pi.pardot.com/user.
2: Create a new user
I like creating a new user dedicated to external integrations, rather than using my own credentials.
Plus, if you’ve set up Pardot to leverage single-sign-on via Salesforce credentials, you can’t even get into the API without having a dedicated Pardot user whose “email address” isn’t the username of a user in the Salesforce org you have connected to Pardot.
New in Summer ‘20: Pardot users connected to Salesforce SSO accounts also have API keys!
You can skip to “5: Get the user key” if you’d like, although I personally still recommend having a whole Salesforce user dedicated to data integrations rather than using the credentials of a real human.
If your company passes through e-mails with “+Something
” on usernames to the username before the “+
,” this is particularly simple – just add something like “+rest
” before the “@
.”
So, for example, your_name+rest@your_company.com
Otherwise, start with an e-mail address that you control but that isn’t a user in the Salesforce org you have Pardot connected to.
(Note: once you get this new user’s password set up, you can change its e-mail address to a fake e-mail address, but you’ll have to start with a real e-mail address so you can get the activation e-mail.)
In the upper-right corner of the “Users” page, click the “Add User” button.
Give the user a descriptive first name & last name. I like “REST” as the first name and “API User” as the last name.
Give the user an e-mail address where you can receive e-mail. If you have Pardot set up to let you log into it using Salesforce credentials, make sure that the e-mail address is not in use as a Salesforce username for whatever org you connected to Pardot.
Give the user a Role of “Administrator” (warning: it can now do some pretty powerful stuff – be careful with its password!).
Un-check all the boxes in “Email Preferences.”
Click the “Create user” button.
If you have Salesforce sign-on turned in, ignore all prompts from Pardot to choose a “CRM Username” for the user you just created.
3: Set a password
Check your e-mail. Open the “activation” link in a new incognito window, or a different browser.
Set a password and a security question+answer for the new user.
Store these in a safe password manager.
Log into Pardot in the same “incognito” tab or browser with the new credentials to make sure they work.
Log out of Pardot and close the browser window. You’ll never need to log into Pardot’s web console with these special credentials again.
4: Optional: change the e-mail address
If you think you might one day want to use the e-mail address you chose earlier as the username of a Salesforce user, change the Pardot user’s e-mail address now.
The new e-mail address doesn’t have to be validated for it to become the new username of the Pardot user, so you can use an e-mail address that isn’t real.
However, make sure that you change it to something that you know will never accidentally be delivered to anyone else, because this is where “password reset” e-mails would go.
Do not use “@example.com
” like I have done in my screenshots. That’s a huge security vulnerability. (I deleted the user from Pardot as soon as I was done taking screenshots.)
So, for example, you could change it to your_name+rest@your_company.com
.
To do this, back in the Pardot session where you’re logged in as yourself, click the “Edit user” button in the upper-right-hand corner of the user detail page for the user you just created.
Edit the e-mail address to something smart and, at the bottom of the page, click “Save user.”
Ta-da! The e-mail address has been changed. Although you may not be able to get password reset e-mails at the e-mail address you chose, you can always temporarily change the e-mail address back to something you can receive e-mail at.
(Just make sure it isn’t being used as a username in the Salesforce org you have Pardot connected to for single-sign-on, if you have that enabled.)
5: Get the user key
For better or for worse, when you’re logged into Pardot as any system administrator, you can see every user’s “API user key.”
(That’s why you don’t ever need to log into the Pardot web console as your new user.)
Looking at the user detail page for the user you just created, scroll down through the details and find the text next to “API User Key.”
You’ll need this “user key” to log into Pardot through the API.
You could add the user key to your password manager along with the username and password for the account, if you really trust your password manager.
Otherwise, you could log into Pardot as yourself and look up this information every time you want to use the API.
6: Secrets -> Python -> Voilà!
The 3 key pieces of information you need to log into the Pardot API with code as a given user are the e-mail address (which functions as a username), the password, and the API User Key.
Open up an IDE from which you can execute Python and run the following script, making appropriate substitutions for “PUTUSERKEYHERE
,” “PUTEMAILADDRESSHERE
,” and “PUTPASSWORDHERE
.”
import requests, urllib
ukey = 'PUTUSERKEYHERE'
api_key = requests.post(
'https://pi.pardot.com/api/login/version/3'
, params='email='+urllib.parse.quote('PUTEMAILADDRESSHERE')
+'&password='+'PUTPASSWORDHERE'
+'&user_key='+ukey
+'&format=json'
).json().get('api_key')
print(api_key)
If everything goes well, print(api_key)
should show you the token you just generated.
For the next 60 minutes, you’ll prove your identity by passing this “session token” value, stored in “api_key
,” alongside the user key, stored in “ukey
,” and various other commands, to one of the Pardot API “endpoints” that do useful things.
Here is official documentation about the HTTPS POST to make when authenticating against Salesforce Pardot’s “API version 3,” which is what the code above logs into.
Note: If you’re using an IDE that saves the contents of variables from one script-run to the next, you can now comment out the entire “authentication” block of code (surround it with “'''
” before the block of code and again after it) and flip the contents back to “PUTUSERKEYHERE
,” “PUTEMAILADDRESSHERE
,” and “PUTPASSWORDHERE
.”
- Q: Why would you do this?
- A: For the same reason it’s a good idea to clean the kitchen as you cook. The thought “password” is fresh in your mind. Now is as good a time as any to make sure you don’t accidentally save code with passwords in it to your hard drive.
Tip: Don’t accidentally execute your code a second time until you’ve commented out ukey = 'PUTUSERKEYHERE'
… you’re going to need the real user key stored in ukey
for subsequent API calls.
That’s it – enjoy programming against Pardot.